JavaScript scope is a fundamental concept that determines the accessibility of variables and functions within your code. Understanding scope is essential for writing clean, organized, and error-free JavaScript.
What is Scope?
Scope defines the region of code where a variable or function can be accessed. In JavaScript, there are four types of scope:
Global Scope: Variables and functions declared outside of any function or block have global scope. They can be accessed from anywhere within the JavaScript code.
Function Scope: Variables and functions declared within a function have function scope. They are only accessible within that function and its nested functions.
Block Scope: Variables declared within a block (using let
or const
) have block scope. They are only accessible within that block and any nested blocks.
Lexical Scope: JavaScript has lexical scope, which means that the scope of a variable or function is determined by its position in the code, not by where it is called.
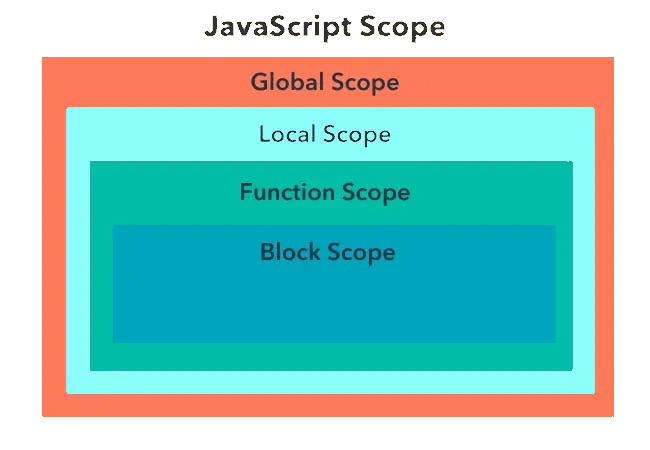
What is JavaScript Scope?
In JavaScript, the concept of scope is one of the most fundamental principles that dictates how variables are accessed and manipulated throughout a program.
Understanding scope is essential for writing efficient, bug-free code. Scope defines the accessibility of variables, functions, and objects in different parts of your Code.
This accessibility is critical because it ensures that variables are kept within the appropriate context and that they don’t interfere with other parts of your code unintentionally.
In this article, we’ll dive deep into JavaScript scope, examining the following topics:
- What is scope in JavaScript?
- Global scope
- Local scope
- Function scope
- Block scope
- Lexical scope
- Wrapping up JavaScript scope
By the end, you’ll have a thorough understanding of how scope operates in JavaScript and how to effectively manage it in your code.
Understanding JavaScript Scope
To put it simply, scope refers to the context or environment in which variables are defined and can be accessed.
When you declare a variable in JavaScript, that variable exists within a specific scope, and that scope determines where you can access or use the variable in your code.
JavaScript has two major types of scope:
- Global Scope: Variables declared outside of any function or block are accessible throughout the entire program, making them available in all parts of your code.
- Local Scope: Variables declared within functions or blocks are limited to that specific context, meaning they cannot be accessed from outside their containing function or block, ensuring encapsulation and preventing unintended interactions.
But beyond these broad categories, we can further classify scope into different types, each affecting how and where variables are accessible in the code. These types include:
- Function Scope
- Block Scope
- Lexical Scope
Before we explore these types in detail, let’s clarify why scope matters. Variables should be managed in such a way that they don’t conflict with each other.
Mismanaging scope can lead to errors such as unintended variable overwriting, difficulty in debugging, and variables leaking into unintended parts of the codebase.
Global Scope
The global scope is the outermost scope in JavaScript. Any variable declared in the global scope is available throughout the entire program, across all functions, blocks, or objects.
How to Declare a Global Variable
In JavaScript, a variable is in the global scope if it’s declared outside of any function or block. Here’s an example:
let globalVar = "I'm global";
function checkScope() {
console.log(globalVar); // Accessible here
}
checkScope(); // Output: I'm global
console.log(globalVar); // Output: I'm global
In the example above, globalVar
is declared in the global scope, so it’s accessible both inside and outside of the checkScope()
function.
Global Scope Issues
While global variables may seem convenient because they are accessible everywhere, they can lead to several issues:
- Naming Collisions: Global variables are accessible from any part of your program, which creates a significantly higher risk of inadvertently overwriting or unintentionally changing variables that share the same name.
This can lead to unexpected behavior in your code, making it hard to track down which variable is being referenced or altered.
. - Difficult Debugging: If you modify global variables in various parts of your codebase, it can become incredibly difficult to pinpoint exactly where the issue lies when debugging errors.
The spread of global variables means that any change can ripple through your application, leading to confusion and wasted time while you search for the source of the problem.
. - Memory Leaks: Since global variables persist for the entire lifetime of your program, they remain in memory longer than necessary.
This long-term retention of global variables can potentially cause memory leaks if not managed properly, consuming valuable resources and affecting the performance of your application over time.
.
Thus, using global variables should be minimized whenever possible. Instead, we should prefer local variables (within specific scopes) to keep the scope of variables manageable and to reduce unintended side effects.
Local Scope
Local scope refers to variables that are accessible only within a specific part of the code—usually within a function or a block. Variables declared within this local scope are not accessible outside of their defined area.
How to Declare a Local Variable
To declare a local variable, you can use let
, const
, or var
inside a function or block. These variables will not be accessible outside of the function or block. Here’s an example using local scope within a function:
function localScopeExample() {
let localVar = "I'm local";
console.log(localVar); // Accessible here
}
localScopeExample(); // Output: I'm local
console.log(localVar); // Error: localVar is not defined
In this example, localVar
is declared inside the localScopeExample()
function, making it accessible only within that function. Attempting to access localVar
outside of the function results in an error.
Benefits of Local Scope
- Avoid Naming Collisions: Variables declared in the local scope do not interfere with other variables in the global scope or in different local scopes.
This isolation helps prevent unintended consequences from variable overwrites and makes the code more predictable, as developers can rely on variable names to function as intended without worrying about conflicts.
. - Memory Management: Local variables exist only for the duration of their enclosing scope (i.e., a function or block).
Once the function or block completes execution, the memory that was allocated for those variables can be freed, allowing for efficient use of system resources. This temporary allocation minimizes memory usage, which can lead to better performance overall.
. - Encapsulation: Local scope plays a crucial role in encapsulating logic and variables within specific blocks of code. This organization not only aids in managing the code’s behavior but also improves readability and maintainability.
By keeping related code and data together, developers can create modular components that are easier to understand and modify, leading to cleaner code structures.
.
Function Scope
Function scope is a specific kind of local scope that applies to variables declared inside functions. Variables declared with var
inside a function are scoped to that function. This means they are accessible only within the function they are declared in and are not accessible outside.
Example of Function Scope
function myFunction() {
var functionScopedVar = "I'm scoped to this function";
console.log(functionScopedVar); // Accessible here
}
myFunction(); // Output: I'm scoped to this function
console.log(functionScopedVar); // Error: functionScopedVar is not defined
In this example, functionScopedVar
is declared with var
inside myFunction
. As a result, it’s accessible only within the function and not outside it.
Hoisting in Function Scope
One of the quirks of var
in function scope is hoisting. JavaScript “hoists” variable declarations to the top of the function, meaning that you can access a variable before it’s declared within the function scope.
function hoistingExample() {
console.log(hoistedVar); // Output: undefined (because of hoisting)
var hoistedVar = "This is hoisted";
}
hoistingExample();
In this example, JavaScript hoists the declaration of hoistedVar
to the top of the function but not its assignment. As a result, it prints undefined
instead of throwing an error.
However, let
and const
do not experience the same hoisting behavior as var
in function scope, making them safer for use in modern JavaScript.
Block Scope
Block scope is a newer addition to JavaScript and comes into play with the introduction of let
and const
in ES6. A block is any code enclosed in curly braces {}
, such as in if
, for
, or while
statements.
Variables declared with let
or const
are scoped to the nearest enclosing block, while var
does not respect block scope and continues to be function-scoped.
Example of Block Scope
if (true) {
let blockScopedVar = "I'm block-scoped";
console.log(blockScopedVar); // Accessible here
}
console.log(blockScopedVar); // Error: blockScopedVar is not defined
In this example, blockScopedVar
is declared inside an if
block using let
, so it’s accessible only inside that block. Trying to access it outside the block will throw an error.
var
and Block Scope
If we used var
instead of let
in the above example, the behavior would be different:
if (true) {
var notBlockScopedVar = "I'm not block-scoped";
console.log(notBlockScopedVar); // Accessible here
}
console.log(notBlockScopedVar); // Accessible here too
Here, notBlockScopedVar
is declared with var
inside the if
block, but because var
does not respect block scope, the variable is accessible outside the block.
Lexical Scope
Lexical scope, also known as static scope, refers to how a function’s scope is determined by its position in the source code.
In JavaScript, the scope of variables is determined during the compile time, based on the location where the function is defined.
This means that inner functions have access to variables of their outer functions due to the way scopes are nested.
Example of Lexical Scope
function outerFunction() {
let outerVar = "I'm from the outer scope";
function innerFunction() {
console.log(outerVar); // Accessible here because of lexical scope
}
innerFunction();
}
outerFunction(); // Output: I'm from the outer scope
In this example, innerFunction()
can access outerVar
because it is lexically within the scope of outerFunction()
. Even though outerVar
is declared outside of innerFunction()
, it is available inside due to lexical scoping.
Closures and Lexical Scope
Closures are an advanced feature of JavaScript that leverage lexical scope. A closure occurs when an inner function retains access to the outer function’s variables even after the outer function has returned.
function outerFunction() {
let outerVar = "I'm still accessible";
return function innerFunction() {
console.log(outerVar); // Retains access to outerVar
};
}
const closure = outerFunction();
closure(); // Output: I'm still accessible
In this case, innerFunction()
retains access to outerVar
even after outerFunction()
has finished executing, demonstrating the concept of closures.
Wrapping Up JavaScript Scope
Understanding JavaScript scope is essential for writing clean, maintainable code. Scope determines where variables can be accessed and manipulated in your program, and mismanaging scope can lead to errors and confusion.
To summarize:
- Global Scope: Variables declared in the global context are accessible anywhere in the code, making them convenient for certain tasks.
However, this widespread accessibility can lead to significant issues, such as naming collisions, where two or more variables share the same name, resulting in unexpected behavior.
Additionally, global variables persist for the entire lifetime of the program, which can lead to memory leaks if they are not properly managed or released when no longer needed.
. - Local Scope: Variables declared within functions or blocks are confined to those specific areas, meaning they cannot be accessed from outside.
This limitation makes local variables safer and more manageable since they help prevent unintended interference with other parts of the code. By keeping variables local, developers can reduce complexity and improve code readability.
. - Function Scope: Variables declared within functions (using
var
) are function-scoped, meaning they are accessible only within the function itself.
This encapsulation helps to organize code logically and prevents external code from inadvertently modifying the internal state of the function. Understanding function scope is crucial for maintaining clean and effective code.
. - Block Scope: With the introduction of
let
andconst
in ECMAScript 6, JavaScript now supports true block-level scope, which limits variables to the specific block in which they are declared.
This improvement allows developers to create more predictable and controlled code structures, especially in loops and conditional statements. The distinction between block scope and function scope helps to further minimize the risk of variable collisions.
. - Lexical Scope: Functions are lexically scoped, meaning they can access variables in the outer scopes based on where they are written, even after the outer function has returned, thanks to closures.
This behavior allows inner functions to retain access to their outer function’s variables, making it possible to create powerful patterns in JavaScript. Lexical scope is foundational for understanding how closures work and plays a vital role in functional programming techniques.
.
By understanding these scope principles, you can write more robust and bug-free JavaScript code that behaves as expected.